Connecting to a Service
Connecting to a Service
Introduction
An admin app ends up losing its meaning if it cannot consume data. So, in the last step of the course, we will learn how to connect your admin app to a service and then use it in the front-end of the application.
Activity
- Start by adding the required builders for service:
/manifest.json
{
...
"builders": {
"react": "3.x",
"messages": "1.x",
"docs": "0.x",
"admin": "0.x",
+ "node": "6.x",
+ "graphql": "1.x"
}
}
- Create a
graphql/
folder and add a simpleschema.graphql
file with a single query:
/graphql/schema.graphql
type Query {
helloworld: String
}
- Create a
node/
folder and add anindex.ts
file to it that will instantiate our service:
/node/index.ts
import { Service } from '@vtex/api'
export default new Service({
graphql: {
resolvers: {
Query: {
helloworld: () => `Service number: ${Math.random()}`,
},
},
},
})
Our service will then, in the query helloworld
, return a random number.
- In the
/node
folder so that you can develop well locally, you will need apackage.json
, you can add a simple one:
/node/package.json
{
"dependencies": {
"co-body": "^6.0.0",
"ramda": "^0.25.0"
},
"devDependencies": {
"@types/co-body": "^0.0.3",
"@types/jest": "^24.0.18",
"@types/node": "^12.0.0",
"@types/ramda": "types/npm-ramda#dist",
"@vtex/api": "6.36.2",
"@vtex/test-tools": "^1.0.0",
"tslint": "^5.14.0",
"tslint-config-vtex": "^2.1.0",
"typescript": "3.8.3"
},
"scripts": {
"lint": "tsc --noEmit --pretty && tslint -c tslint.json --fix './**/*.ts'"
},
"version": "0.0.7"
}
- In the
react/
folder we will need to define a query to be able to use the resolver that we defined in the service. To do this, create agraphql/
folder inside thereact/
folder and in this folder, create ahelloworld.gql
with:
/react/graphql/helloworld.gql:
query hello {
helloworld
}
- Finally, we need to add this query to our component in
adminExample.ts
import React, { FC } from 'react'
import { Layout, PageBlock } from 'vtex.styleguide'
+import { useQuery } from 'react-apollo'
+import helloworld from './graphql/helloworld.gql'
const AdminExample: FC = () => {
+ const { data } = useQuery(helloworld)
return (
<Layout>
<PageBlock title="Title" subtitle="Some explanation." variation="full">
<h1>Hello, World!</h1>
+ <p>{data?.helloworld}</p>
</PageBlock>
</Layout>
)
}
export default AdminExample
The result should be:
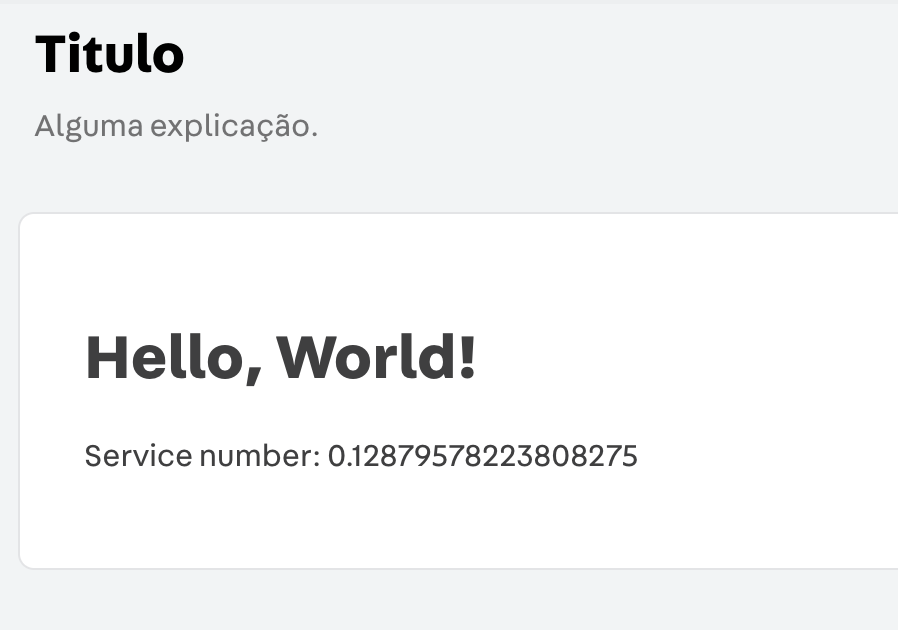
With this you have a service connected to your admin application and can now connect to external services (learn more about our services course 🚀).
Any questions?
See the answersheet for this step or check our office hours on the VTEX Developers channel.
Help us make this content better!
VTEX IO courses are open source. If you see something wrong, you can open a pull request!
Make a contribution
Updated about 1 month ago